What is prototyping?
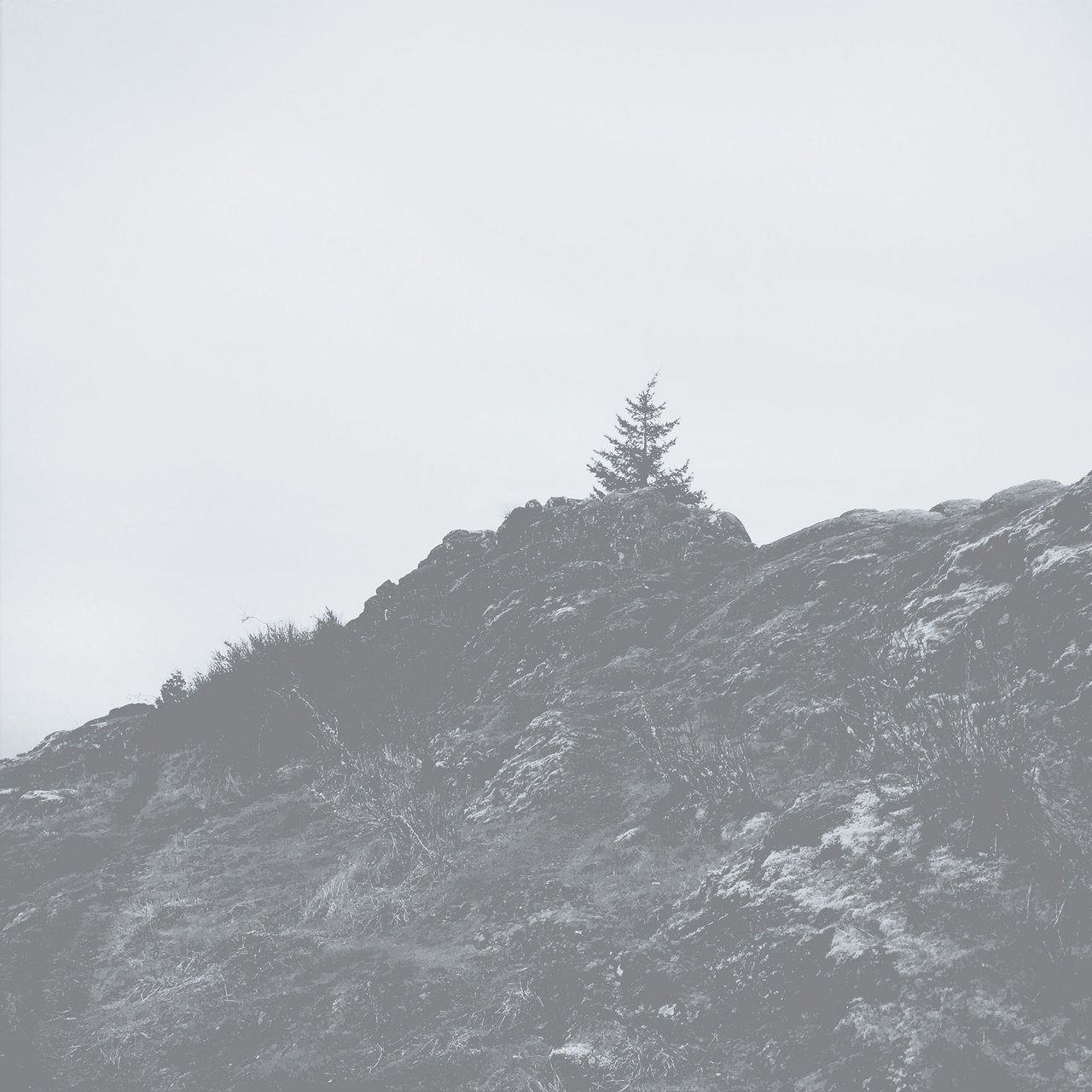
You have an idea for a new online product offering. Maybe you already have a thriving business and you
want to reach more customers with an interactive experience. Or maybe you're a budding
entrepreneur who has a fresh idea to take to market.
What's the first thing that you should do?
Maybe you should brainstorm a compelling and memorable name. Then go register a domain name.
Hire a designer to create a logo.
Sketch some wireframes.
Hire a developer.
Build the app.
These all sound like reasonable starting points. And they're all things thriving product businesses have done,
so it's natural you'd follow this progression.
But none of these activities involve the reason you're building your product in
the first place: your customers.
Your customers aren't going to come to you because of your name.
They're not going to pay you because you have a sweet logo.
And they don't care about your app.
Your customers only care about one thing: That their life is
improved because your product is in their lives.
It's fair to say then that your customers care about outcomes. They care that,
after using your product, they feel better, have more money, or are otherwise
more satisfied than before using your product.
An outcomes-oriented approach
Instead of focusing on deliverables like mockups, technology, and a snazzy marketing page,
what if you focused on the outcomes your customers will experience? What if you
spent time answering questions like...
- Who am I serving?
- What problem am I solving for them?
- What makes me better than the competition?
The answers to these questions might involve technology or visual design, if
you decide those things are necessary to helping your customers solve their
problem. But they certainly aren't the core reason your customers want what
you've got. You've got to identify why they came to you in the first place. How
do they think? What annoys them? How can you alleviate their existential
suffering... or at least make their lives marginally easier?
When you know who you're serving, you're able to learn from them about what
they want. And when you know the problem you're solving and how your solution is
unique, you won't dilute your message and scope.
If you're actually solving someone's problem, they won't care if your branding
isn't perfect. They won't mind if your site looks terrible on mobile, so long as
they don't need to use the site on mobile. And even if they do: As long as
they're getting value from your product, they'll be willing to wait.
That's the essence of prototyping: Building a working version of a product
idea that provides real value to real human people, and putting everything else
off until after you've done that.
Your objective is to confirm or refute your product's value hypothesis
by conducting experiments which simulate aspects of your product idea—not to
hold off "launching" your product until a "public release."
That doesn't mean you won't eventually choose a marketable name. It doesn't mean you're
going to skimp on any aspect of your product for good. It just means you
recognize that the thing your product must do in order to be viable is provide
someone somewhere with real value.
If you're doing that, you have a successful
product. If you're not, you just have a bunch of code and images and text
chasing a fantasy.
The prototyping process
I've spent my career leading the early stages of digital products.
Here's the process I use to reduce cost and encourage experimentation when
taking a new product to market:
1. Identify the problem you're solving, and for whom
Clearly define the problem your new product alleges to solve, and the specific
segment of the market who has the problem.
My favorite exercise for defining your problem and target market is the
Fool-Proof Positioning Statement by Dan Janal:
The Fool-Proof Positioning Statement is a two-sentence message that tells people
what your product is and how they will benefit. The second sentence tells people
why your product is different than others. Here's an example: David Letterman is
a talk show host who entertains
baby boomers so they can feel good before they go to bed. Unlike other talk show
hosts, he performs a Top Ten List.
A positioning statement identifies the following elements:
- Category of product
- Primary audience
- Primary benefits
- Competing products
- Primary difference or uniqueness
Take for example, a positioning statement for Formbot,
my SaaS for sending webform submissions to Slack and email:
Formbot is an online service that helps developers of static sites receive
feedback from their visitors without setting up a server. Unlike other form
services, Formbot connects to Slack.
I had the idea for Formbot because I had a real and annoying problem: I love building
websites with static site generators, but I didn't
want to have to set up a server just to receive form feedback from my visitors.
Once you've identified your primary audience, identify real people within that
audience who have the problem you want to solve. Get their assurance that they
would gladly pay money to have the problem solved. Forge relationships with
them. Ask them how your competitors' product could be better. Listen.
2. Identify desired outcomes from using your product
Now that you have a handle on the problem and the audience for whom you're solving it,
it's time to identify how you're going to solve their problem.
Do your users want a fully-automated solution, or one with an interface that
affords more customizability?
What are your audience's success outcomes? If your audience gets nothing else
out of using your product, what is the one thing with which they need to leave
in order to continue using the product?
Organize your product's hypothetical features into user stories. These are a
special type of device for thinking about a product's features in terms of their
outcomes instead of their deliverables. Each well-written user story identifies a
persona, action, and outcome for a given software feature:
As a Formbot user, I want to connect my Slack account so that I can receive
potential sales leads in Slack.
-
As a...: the persona who has a stake in using the product
-
I want to...: the action they're going to perform to reach a desired
outcome
-
so that...: the valuable outcome the product grants them
Note that the action for each user story needn't be explicit. You don't need
to explain that your user should press the "Create Message" button; just explain
that they're going to send a message and why that's important. For instance,
here's an example of a user story that's too bound to deliverables and has no
real business outcome:
As a user, I want to click the "Create Message" button to open the Create
Message dialog so that I can send a message to my clients.
Instead, focus on the outcome of the action as it relates to the user:
As a user, I want to send a message to my clients so that I can follow up with
them and make more sales.
In doing so, you decouple implementation from your outcomes. When you engage a
developer to build the first version of your product, you'll be measuring
success not by whether there's a button that reads "Create Message" (irrelevant
to your business), but by whether your users can effectively reach their clients
(relevant to your business).
3. Determine the most valuable feature of the solution
Of all the user stories you wrote, which one offers the most value to your
users? If you were stranded on a desert island and your developer could only
build one feature (yes this is a terrible analogy), which feature would you have
them build?
Do your prospective users from step 1 feel the same way? Would they start using
your product if you could deliver them that one feature?
Formbot started out as a single feature with hardly any user interface. It was
barebones, but it did one thing exceptionally well. So it attracted a small but
loyal userbase. As a result, I was able to capture user feedback and better
understand why users were satisfied and why they weren't. This informed further
development and further feedback collection.
You might think you need features that most products have, like
email alerts or two-factor authentication. But when you force yourself to think in terms
of delivering value to real human people you are actually talking to right
now, you find ways to help them without a ton of expensive engineering work.
Again: That's not to say email alerts and two-factor authentication aren't
valuable. They're both immensely valuable and you should build them. They
might even be inseparable from your most valuable feature, and you might need
to build them in order to satisfy your users. But strive to build the least
product possible to deliver the most value. Usually that's less than you think.
4. Build and deliver that feature to your audience
Now that you know the featureset that will deliver the most value to your users,
it's time to build it.
It's not time to create wireframes. Nor is it time to hire a designer. These are
both actions that result in deliverables, like wireframes and mockups. We're prototyping, so we don't want
deliverables. We want outcomes.
The first iteration of your product probably won't be pretty. But it'll solve a
real problem that your identified real human users need solved. So it doesn't
need to be pretty. It needs to work. And for that, you need a developer.
Developers are a dime a dozen. Most developers focus on deliverables. They build
the features you want built in the way you specify. They paint by numbers.
What you want is a developer who focuses on outcomes. Remember the user stories
you wrote in step 2? You know how I told you to make sure they specify your
desired outcomes, as opposed to the path for getting there? You want to find a
developer who thinks like that. Someone who sees the road ahead of your business
and can steer your product accordingly.
Instead of asking how? like most developers, you want to find a developer who asks
why? Instead of estimating how long it'll take to deliver "your app," you want
to find a partner who can estimate how long it'll take to validate your
business.
Here's a secret truth about software development no one wants you to know:
You're never done. Your product will always have bugs, unimplemented features,
or things you don't like. It's a fact of the business.
Developers don't want you to know this because they
make their money on deliverables. For them to be done with a project is for all the
deliverables to be completed. But by this definition of completeness, no project
is ever finished.
But you're not going to focus on deliverables. You're going to focus on
outcomes. You know that by focusing on deliverables, you can't clearly say
whether you've achieved your desired outcome. Having a product with a gorgeous
user interface that doesn't have any users is a sure way to go broke.
But having a product with an ugly interface that helps 1,000 people achieve a
desired outcome means you've proven your hypothesis. The gorgeous interface will
come later.
5. Assess the value delivered
You've built your first feature. Your users are
now able to walk through one workflow from start to finish. It's not pretty, but it works.
Now it's time to see how your audience responds.
Because of how little you've actually built, you might feel ashamed to share
this with your audience. But ask yourself this: How would you feel if you made
it "perfect", shared it with your audience, and discovered it didn't help them?
And what about the best case scenario? What if your one feature helps your
audience in ways you didn't think it could? If that happens, then
congratulations! You've validated your product idea. And you did it without
spending all your money on vanity deliverables like branding, visual design, and SEO marketing.
6. Refocus your efforts accordingly
If your first feature was a hit, then you're probably feeling encouraged and want to press on.
If your audience didn't respond the way you hoped, then you're
probably a bit discouraged and might feel like giving up.
Whatever happened, you can rest assured that because you reduced your
deliverables to only those things that help you validate whether you can deliver your
users the outcomes they desire, 100% of your investment was in pursuit of
providing your users with a valuable experience.
You didn't spend lavishly on a hip domain name.
You didn't hire an expensive visual designer.
And hopefully, your sunk cost is low enough that you have the emotional
wherewithal to be able to walk away unscathed.
Or, you can choose to regroup and reposition your product. Because you didn't
lock yourself into a specific featureset with lavish marketing and polish, you
can conduct another experiment. You can continue this process indefinitely until
you find something that sticks.
That's the beauty of prototyping. That's why I focus on outcomes. And that's how
to build a product people will actually use.
Read on: How is prototyping different?